Quicksort Algorithm
Quicksort Algorithm
Quicksort is an algorithm based on divide and conquer approach in which the array is split into subarrays and these sub-arrays are recursively called to sort the elements.
How QuickSort Works?
- A pivot element is chosen from the array. You can choose any element from the array as the pviot element.
Here, we have taken the rightmost (ie. the last element) of the array as the pivot element. - The elements smaller than the pivot element are put on the left and the elements greater than the pivot element are put on the right.
The above arrangement is achieved by the following steps.- A pointer is fixed at the pivot element. The pivot element is compared with the elements beginning from the first index. If the element greater than the pivot element is reached, a second pointer is set for that element.
- Now, the pivot element is compared with the other elements. If element smaller than the pivot element is reached, the smaller element is swapped with the greater element found earlier.
- The process goes on until the second last element is reached.
- Finally, the pivot element is swapped with the second pointer.
- A pointer is fixed at the pivot element. The pivot element is compared with the elements beginning from the first index. If the element greater than the pivot element is reached, a second pointer is set for that element.
- Pivot elements are again chosen for the left and the right sub-parts separately. Within these sub-parts, the pivot elements are placed at their right position. Then, step 2 is repeated.
- The sub-parts are again divided into smallest sub-parts until each subpart is formed of a single element.
- At this point, the array is already sorted.
Quicksort uses recursion for sorting the sub-parts.
On the basis of Divide and conquer approach, quicksort algorithm can be explained as:
- Divide
The array is divided into subparts taking pivot as the partitioning point. The elements smaller than the pivot are placed to the left of the pivot and the elements greater than the pivot are placed to the right. - Conquer
The left and the right subparts are again partitioned using the by selecting pivot elements for them. This can be achieved by recursively passing the subparts into the algorithm. - Combine
This step does not play a significant role in quicksort. The array is already sorted at the end of the conquer step.
You can understand the working of quicksort with the help of an example/illustration below.
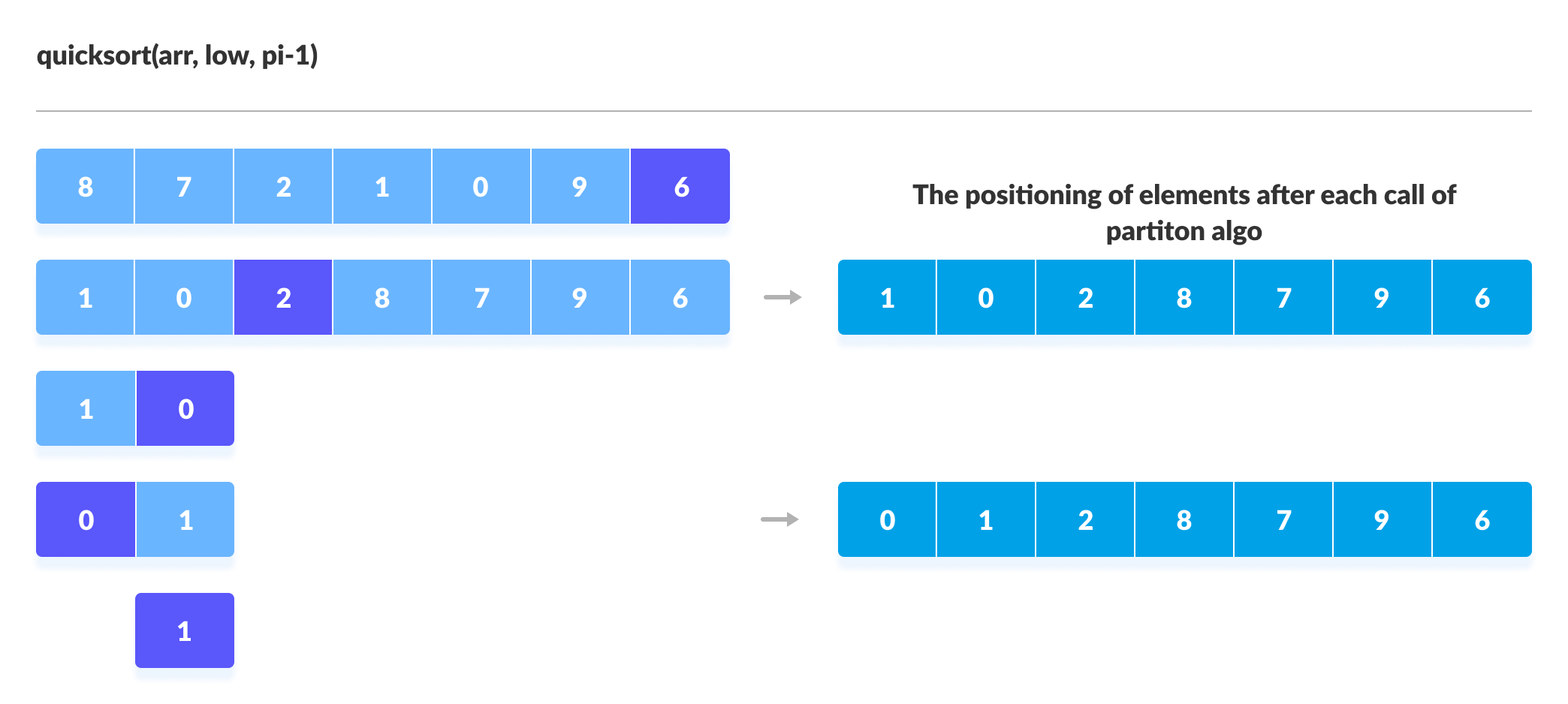
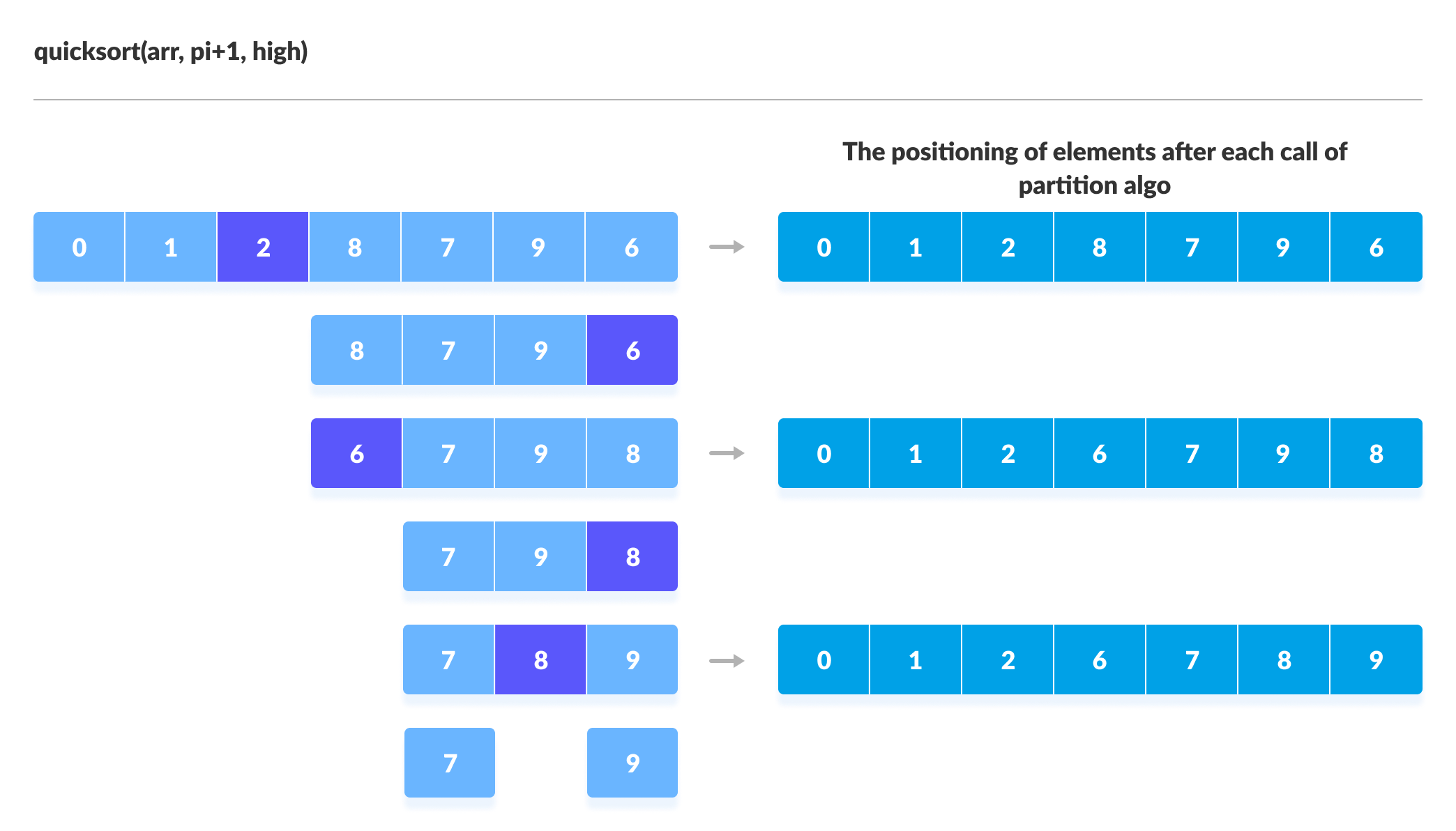
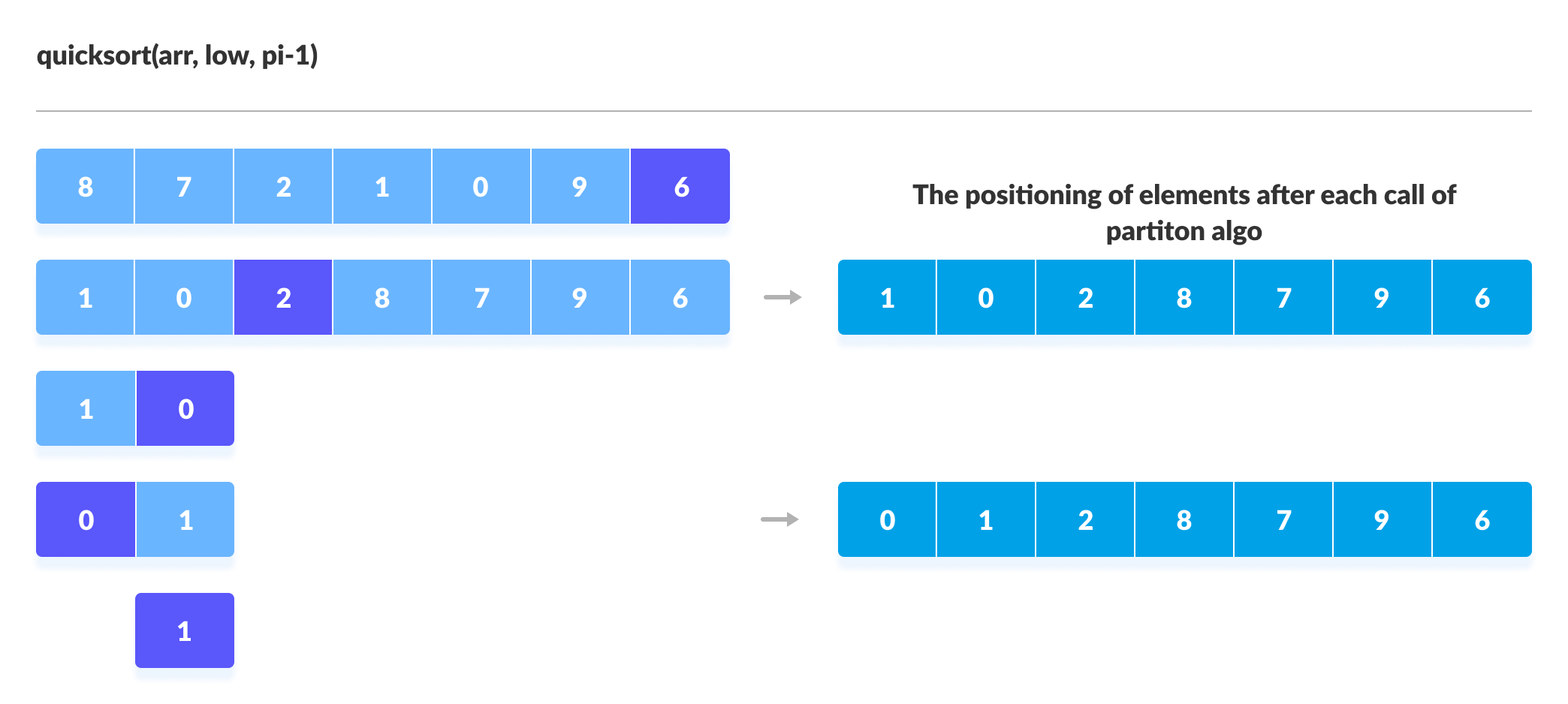
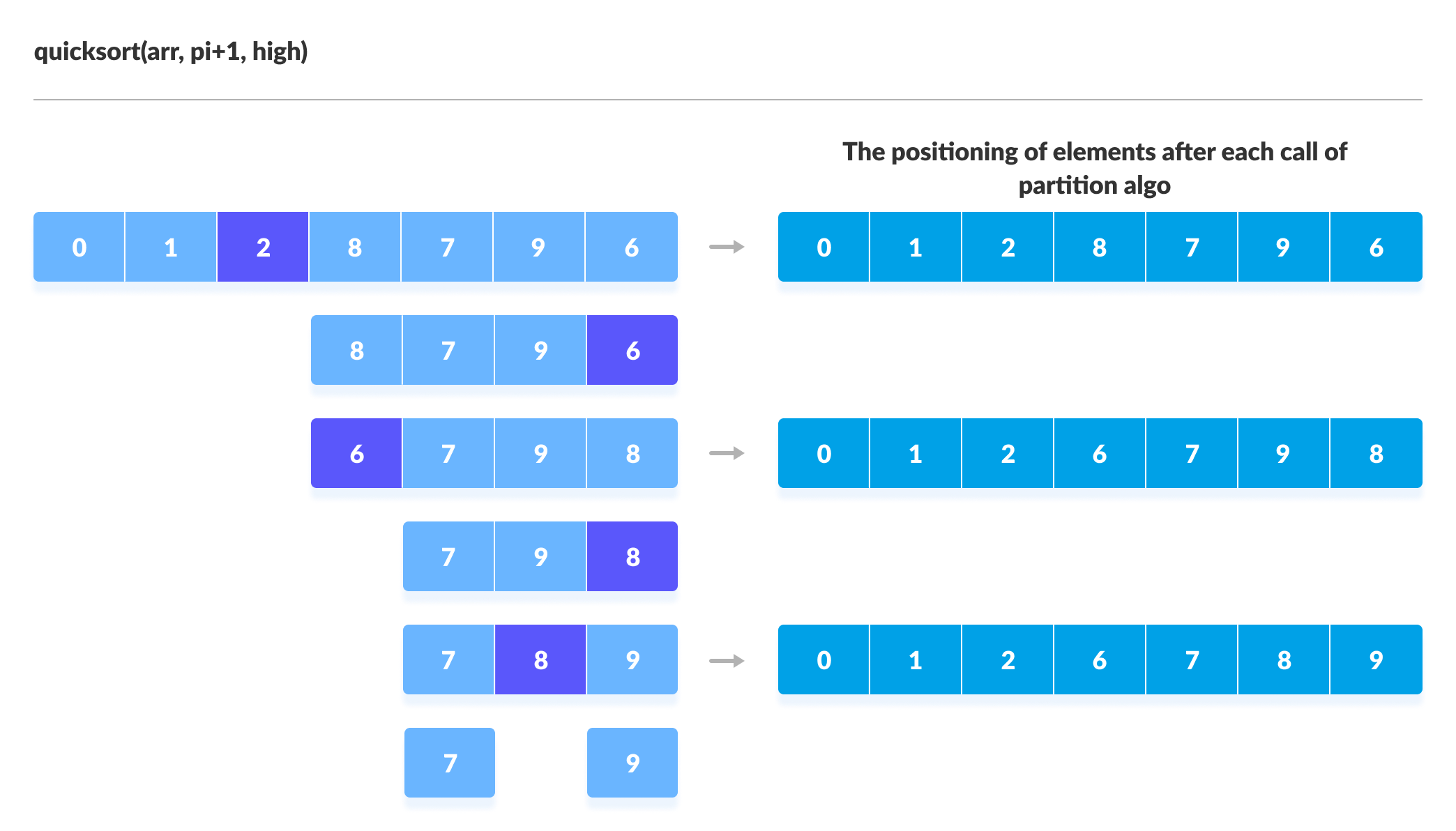
Quick Sort Algorithm
quickSort(array, leftmostIndex, rightmostIndex)
if (leftmostIndex < rightmostIndex)
pivotIndex <- partition(array,leftmostIndex, rightmostIndex)
quickSort(array, leftmostIndex, pivotIndex)
quickSort(array, pivotIndex + 1, rightmostIndex)
partition(array, leftmostIndex, rightmostIndex)
set rightmostIndex as pivotIndex
storeIndex <- leftmostIndex - 1
for i <- leftmostIndex + 1 to rightmostIndex
if element[i] < pivotElement
swap element[i] and element[storeIndex]
storeIndex++
swap pivotElement and element[storeIndex+1]
return storeIndex + 1
C++ Examples
// Quick sort in C++
#include <iostream>
using namespace std;
void swap(int *a, int *b)
{
int t = *a;
*a = *b;
*b = t;
}
void printArray(int array[], int size)
{
int i;
for (i = 0; i < size; i++)
cout << array[i] << " ";
cout << endl;
}
int partition(int array[], int low, int high)
{
int pivot = array[high];
int i = (low - 1);
for (int j = low; j < high; j++)
{
if (array[j] <= pivot)
{
i++;
swap(&array[i], &array[j]);
}
}
printArray(array, 7);
cout << "........\n";
swap(&array[i + 1], &array[high]);
return (i + 1);
}
void quickSort(int array[], int low, int high)
{
if (low < high)
{
int pi = partition(array, low, high);
quickSort(array, low, pi - 1);
quickSort(array, pi + 1, high);
}
}
int main()
{
int data[] = {8, 7, 6, 1, 0, 9, 2};
int n = sizeof(data) / sizeof(data[0]);
quickSort(data, 0, n - 1);
cout << "Sorted array in ascending order: \n";
printArray(data, n);
}
Time Complexities
- Worst Case Complexity [Big-O]:
O(n2)
It occurs when the pivot element picked is always either the greatest or the smallest element. In the above algorithm, if the array is in descending order, the partition algorithm always picks the smallest element as a pivot element.- Best Case Complexity [Big-omega]:
O(n*log n)
It occurs when the pivot element is always the middle element or near to the middle element.- Average Case Complexity [Big-theta]:
O(n*log n)
It occurs when the above conditions do not occur.Space Complexity The space complexity for quicksort isO(n*log n)
.
Quicksort Applications
Quicksort is implemented when
- the programming language is good for recursion
- time complexity matters
- space complexity matters
Comments
Post a Comment